SQL Server Joins are essential SQL Server actions to aggregate data from two or more tables based on related columns. To help you become an expert in SQL Server data retrieval, this article thoroughly analyzes the various join types, their advantages, possible disadvantages, and real-world applications.
Table of Contents
Introductions
SQL Server Joins are fundamental SQL procedures that enable merging rows from two or more tables according to a shared column. Combining data from several tables creates a more unified dataset that facilitates thorough data analysis and reporting.
A Glimpse into History
Since SQL’s early days in the 1970s, joins have been essential. Joins were first thought of as a way to manage relational databases. Still, they have changed dramatically over time, becoming more effective and powerful with each new version of SQL Server, from the late 1980s Sybase SQL Server to the current Microsoft SQL Server.
Advantages and Disadvantages of SQL Server Joins
The benefits and drawbacks of SQL Server Joins are discussed below, along with an example:
Advantages
Below are some Advantages of SQL Server Joins:
Integration of Data
Comprehensive Data Analysis: By combining data from several tables, joins provide a unified picture of linked data that may be used for more thorough reporting and analysis.
Flexibility in Data Retrieval: You can retrieve and combine data in various ways depending on your particular needs thanks to the many join types (Inner, Left, Right, Full, Cross, and Self join).
Effectiveness
Optimized Queries: Joins can be made more efficient and quickly executed using indexes to retrieve data, mainly when working with massive datasets.
Reduced Data Redundancy: You may guarantee that your database normalization requirements are upheld and that data redundancy is prevented by employing joins.
Integrity in Relationships
Maintains linkages: By utilizing and supporting the foreign critical linkages between tables, joins ensure data integrity and consistency throughout the database.
Better Information Insights
Complex Queries: Joins facilitate the development of intricate queries that provide comprehensive answers to complicated business problems, fostering more in-depth understanding and improved decision-making.
Scalability
Handle Big Datasets: Well-optimized joins can quickly process and handle big datasets, which qualifies them for use in enterprise-level applications.
Disadvantages
The drawbacks of SQL Server Joins are given below: .
Intricacy
Composing Complex Joins: Composing and managing complex join queries can be difficult, particularly for inexperienced users or when handling several tables and relationships.
Debugging: Join query debugging and troubleshooting can be challenging, especially when handling several joins and complex circumstances.
Problems with Performance
Worse Performance: Longer query execution times and worse performance can result from poorly constructed join queries or joins on big, unindexed tables.
Resource-Intensive: Joins have the potential to be resource-intensive, requiring a large amount of CPU and memory, which could affect the SQL Server’s overall performance.
Upkeep
Schema Changes: Modifications to join queries may be necessary in response to changes in table schemas or relationships (such as adding or removing columns), which would raise maintenance costs.
Dependency Management: Managing dependencies among tables can become challenging in dynamic and often changing database setups.
Risks to Data Integrity
Inaccurate Joins: Improper join conditions can produce incorrect results, compromising the correctness and integrity of the data, such as missing data or duplicate rows.
Null Handling: When null values in join conditions are mishandled, it might result in unexpected outcomes or data loss.
Learning Curve
Steep Learning Curve: For new users, grasping the fundamentals of relational databases can be challenging as it requires a thorough comprehension of the various join types.
What does a SQL Server join mean?
In SQL Server, a join is an SQL operation that combines rows from two or more tables according to a shared column. In relational databases, where data is frequently divided across several tables, joins are crucial for accessing the database. You can obtain a comprehensive dataset that combines data from these several tables by utilizing joins.
How SQL Server Joins Work
SQL Server merges columns from the joined tables into a single result set when you execute a join. The join condition establishes the foundation for matching rows from the involved tables and is commonly expressed using the ON clause.
Types of SQL Server Joins
There are various join types that SQL Server provides, and each has a specific function. Inner Join, Left Join, Right Join, Complete Join, Cross Join, and Self Join are the primary types. Let’s examine each category in-depth, providing examples and thorough explanations.
The Inner Join
Rows from an inner join are returned when the values in the two tables match. This join type is the most widely utilized one.
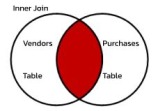
For instance:
Consider that you wish to locate the Purchases from each Vendors has placed. You have two tables: Vendors and Purchases.
-- Script to create Vendors Table
CREATE TABLE Vendors (
VendorID INT PRIMARY KEY,
VendorName NVARCHAR(100),
VendorLocation NVARCHAR(100),
IsActive bit Default 1,
CreatedOn DateTime Default GETDATE()
);
-- Script to create Purchases Table
CREATE TABLE Purchases (
PurchaseID INT PRIMARY KEY,
VendorID INT,
PurchaseDate DATE,
IsActive bit Default 1,
PurchaseAmount DECIMAL(10, 2),
FOREIGN KEY (VendorID) REFERENCES Vendors(VendorID)
);
-- Script to Insert a few sample data into the Vendors table
INSERT INTO Vendors (VendorID, VendorName, VendorLocation)
VALUES
(1, 'TATA STEEL', 'India'),
(2, 'ArcelorMittal', 'Luxembourg'),
(3, 'Ansteel Group', 'China'),
(4, 'Nippon Steel Corporation', 'Japan'),
(5, 'POSCO Holdings', 'South Korea'),
(6, 'Glencore plc', 'Switzerland'),
(7,'Vale','Brazil');
-- Script to Insert a few sample data into the Purchases table
INSERT INTO Purchases (PurchaseID, VendorID, PurchaseDate, PurchaseAmount)
VALUES
(1, 1, '2024-01-15', 2150.00),
(2, 2, '2024-01-20', 2400.00),
(3, 1, '2024-01-10', 2550.00),
(4, 3, '2024-01-15', 3500.00),
(5, 4, '2024-01-05', 6180.00),
(6, 5, '2024-01-05', 580.00),
(7, 2, '2024-01-05', 7180.00),
(8, 3, '2024-01-05', 9180.00),
(9, 2, '2024-01-05', 3180.00),
(10, 5,'2024-01-05', 6830.00),
(11, NULL, '2024-01-15', 100.00),
(12, NULL, '2024-01-05', 100.00),
(13, NULL, '2024-01-20', 100.00),
(14, NULL, '2024-01-25', 100.00);
-- Script to Join both Vendors and Purchases tables & get vendor-wise purchase details
SELECT v.VendorName,
v.VendorLocation,
p.PurchaseID,
p.PurchaseDate,
p.PurchaseAmount
FROM Vendors v
INNER JOIN Purchases p ON v.VendorID = p.VendorID
WHERE v.IsActive=1 AND p.IsActive=1;
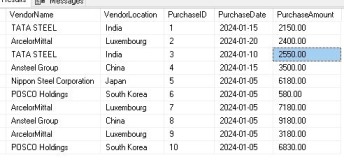
The Left Join
All rows from the left table and any matching rows from the right table are returned by a left join.
if there is no match from right table, it will return NULL values.
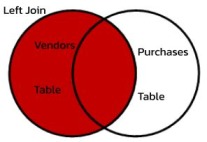
For instance:
A Left Join would be used to locate every Vendors and their Purchases, even those who haven’t placed any purchase.
-- Script to Left Join & locate every Vendors and their Purchases, even those who haven't placed any purchase.
SELECT p.PurchaseID,v.VendorName,
v.VendorLocation,
p.PurchaseDate,
p.PurchaseAmount
FROM Vendors v
LEFT JOIN Purchases p ON v.VendorID = p.VendorID
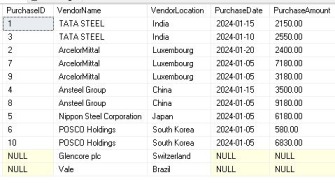
The Right Join
All rows from the right table and matching rows from the left table are returned by a right join. NULL values are returned for the columns in the left table if there is no match.
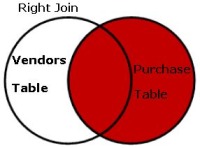
For instance:
Right Join would be used to locate all purchases and their vendors, including purchases without any linked vendors information.
-- Script to Right Join & locate all purchases and their vendors, including purchases without any linked vendors information.
SELECT v.VendorID,
v.VendorName,
v.VendorLocation,
p.PurchaseID,
p.PurchaseDate,
p.PurchaseAmount
FROM Vendors v
RIGHT JOIN Purchases p ON v.VendorID = p.VendorID;
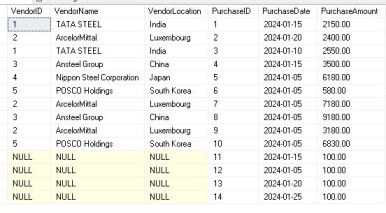
The Full Join
When there is a match in one of the tables, an entire Join returns rows. The outcomes of the Left Join and Right Join are combined.
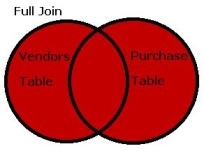
For instance:
You would use a Full Join to obtain a comprehensive list of all vendors and purchases, including those that don’t have matches in the other table.
--Script for Full Join to obtain a comprehensive list of all vendors and purchases, including those that don't have matches in the other table.
SELECT v.VendorID,
v.VendorName,
v.VendorLocation,
p.PurchaseID,
p.PurchaseDate,
p.PurchaseAmount
FROM Vendors v
FULL JOIN Purchases p ON v.VendorID = p.VendorID;
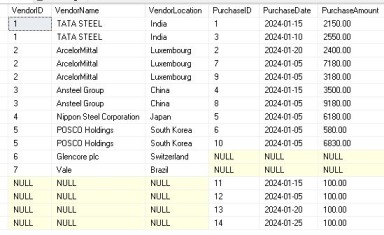
The Cross-Join or Intersection
By merging all of the rows from the first table with all of the rows from the second table, the cross-join method yields the Cartesian product of both tables.
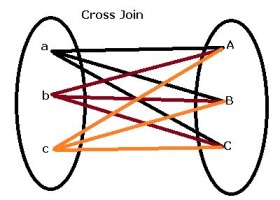
For instance:
A cross-join would be used to get a list of every possible combination of Vendors and Purchases.
SELECT v.VendorID AS VendorID,
v.VendorName AS VendorName,
v.VendorLocation AS VendorLocation,
p.PurchaseID AS PurchaseID,
p.PurchaseDate AS PurchaseDate,
p.PurchaseAmount AS PurchaseAmount
FROM Vendors v
CROSS JOIN Purchases p;
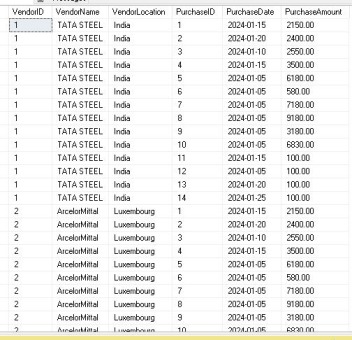
The Self Join
A table can be joined to itself using a self-join. Finding relationships inside a single table or searching hierarchical data are two applications for it.
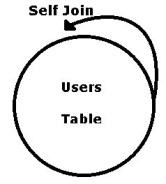
For instance:
You would use a Self Join to locate the Distributors and the corresponding delaers inside an Users table.
-- Script to create the Users table
CREATE TABLE Users (
UserID INT IDENTITY(1,1) PRIMARY KEY,
UserName VARCHAR(100),
UserType VARCHAR(50), -- DL - 'Dealer', DS -'Distributor'
IsActive bit DEFAULT 1,
CreatedOn DateTime DEFAULT GETDATE(),
ParentUserID INT -- References another UserID for relationships
);
go;
-- Script to Insert a few sample records into the Users table
INSERT INTO Users (UserName, UserType, ParentUserID)
VALUES
('DishTV Dealer-A', 'DL', 3),
('DishTV Dealer-B', 'DL', 4),
('DishTV Distributor X', 'DS', null), -- Linked to Dealer A
('DishTV Distributor Y', 'DS', null), -- Linked to Dealer B
('DishTV Distributor Z', 'DS', null), -- Linked to Dealer A
('DishTV Dealer-C', 'DL', 5),
('DishTV Dealer-D', 'DL', 3),
('DishTV Dealer-E', 'DL', 3),
('DishTV Dealer-F', 'DL', 3),
('DishTV Dealer-G', 'DL', 5),
('DishTV Dealer-H', 'DL', 5);
go;
-- Script to Self join the Users tables to get dealer and distributor details
SELECT dl.UserID AS DealerID,
dl.UserName AS DealerName,
ds.UserID AS DistributorID,
ds.UserName AS DistributorName
FROM Users dl
JOIN Users ds ON ds.UserID = dl.ParentUserID
WHERE dl.UserType = 'DL'
AND ds.UserType = 'DS';
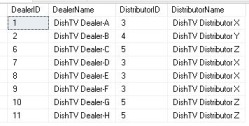
When to Use SQL Server Joins
SQL Server Joins, which let you aggregate rows from two or more tables based on similar columns, are crucial tools in SQL Server for data retrieval. The following are the main situations and applications in which joins in SQL Server should be used:
1. Merging Information from Several Tables using SQL Server Joins
Situation: You need to get a complete dataset that contains information from all of the tables in which your data is dispersed.
2. Preserving Relationships and Data Integrity
Scenario: In a relational database, relationships between tables are specified. You must use and enforce these relationships, including foreign key constraints.
3. Obtaining Information for Reports using SQL Server joins
Scenario: You need data from several sources to create reports.
4. Data Filtration Using Related Tables
Situation: Data in one table needs to be filtered according to criteria in another table.
5. Carrying Out Data Analysis and Aggregation
Scenario: You have to work with several tables in aggregate computations.
6. Managing Data Hierarchies with SQL Server Joins
Situation: Within a single table, you must operate with hierarchical data.
7. Transformation and Migration of Data with SQL Server Joins
Scenario: During data migration or ETL procedures, you must convert data from several tables into a single format.
Typical problems with SQL Server joins
Common issue with SQL Server joins are given below for more clarity & better understanding:
1. Inaccurate Conditions for Joining
Problem: When join conditions are misused, unexpected outcomes can occur, like a Cartesian product (all possible row combinations).
Solution: Consistently make sure that the join condition links the tables appropriately. Verify the columns that the ON clause uses twice.
2. Inaccurate or Missing Join Conditions
Problem: A Cartesian product can occur if the join requirement is wholly overlooked.
Solution: The join condition should always be stated in the ON clause.
3. Large Table Performance Issues
Problem: Performance issues arise when joining big tables, particularly if the join columns are not indexed.
Solution: To enhance efficiency, make sure the join columns are indexed. Think about improving the query plan or utilizing query hints.
4. SQL Server Joins Column Null Values
Problem: When using an INNER JOIN, null values in join columns may cause unexpected outcomes or missing matches.
Solution: If you anticipate null values, use the proper join types (such as LEFT JOIN) and handle null values specifically in your query logic.
5. Identical Rows
Problem: When tables with one-to-many relationships are joined without appropriate grouping or filtering, duplicate data may arise.
Solution: To deal with duplicates, use DISTINCT, GROUP BY, or other suitable filtering.
6. Inexplicable Column Titles
Problem: It can confuse if the same name column appears in both tables.
Solution: To prevent confusion, always qualify column names with table aliases.
7. Inaccurate Outer Join Results
Problem: When using outer joins incorrectly, the result set may contain unexpected null values.
Solution: Apply LEFT, RIGHT, and FULL OUTER JOINs appropriately by understanding their semantics.
8. In Complex Queries, Join Order
Problem: The join order might impact the readability and speed of complex queries.
Solution: Employ Common Table Expressions (CTEs) and think about dividing complicated queries into smaller sections in addition to following a logical order.
9. Views Degrading Performance
Problem: Because of the underlying query complexity, joining tremendous views or employing views in complex joins might lead to performance degradation.
Solution: Use indexed views, optimize views, or materialize interim results as needed.
10. A Lot of Tables Joined
Problem: Excessive table joining in a single query can result in intricate execution strategies and poor performance.
Solution: Simplify your queries, use CTEs or subqueries, and ensure correct indexing.
Conclusion
SQL Server joins provide several benefits, including quick querying, relational integrity preservation, and complete data integration. However, they have several drawbacks, such as a steep learning curve, potential performance concerns, and complexity in query writing and maintenance. When deciding when and how to use SQL Server joins effectively in the environments, database professionals can make more informed judgments by being aware of these benefits and drawbacks.
Frequently Asked Questions (FAQ)
Q: Describe an inner join.
Ans: Rows from both of the join’s concerned tables that have matching values are returned by an inner join.
Q. When is a left join appropriate?
Ans: Whether or not there are matching rows in the right table, use a left join when you need every row from the left table.
Q. What are the differences between a left join and a right join?
Ans: The output of a right join in SQL Server is all rows from the right side table and also matching rows from the left side table.
Q. Full Join: What Is It?
Ans: When a match occurs in one of the tables, a Full Join combines the Left and Right Join outcomes to return rows.
Q. How are cross-joins performed?
Ans: A cross-join yields the Cartesian product of the two tables by merging all of the rows from the first table with all the rows from the second table.
Q. What is the purpose of a self-join?
Ans: A self-join is used to join a table to itself to find associations within the same table, such as hierarchical data.
Q. Can more than two tables be combined into one query?
Ans: You can join multiple tables by adding more join conditions to your query.
Q. When employing joins, what performance considerations are there?
Ans: Joins can use many resources, mainly when dealing with big tables. Proper indexing and query optimization are essential to keeping performance high.
Q. If a join has no matching rows, what happens?
Ans: Rows for Inner Joins are not returned. Columns from the table that do not have matching rows are returned with NULL values for Left, Right, and Full join.
Q. How can I write join queries more often?
Ans: To gain experience creating join queries, you can set up sample databases and execute queries to see how various joins impact the output.
Review the below articles also
Decommission A Server: In 6 Easy Steps
Discover Polybase: Top 7 Usage
PAGEIOLATCH_SH: Unveiling the Secrets
DBCC Freeproccache: A powerful command