Effective database management relies heavily on SQL Server functions & types of functions, which let programmers package logic and simplify intricate tasks. Gaining a grasp of these functions is essential for anybody using SQL Server, regardless of experience level, to maximize database performance and guarantee application consistency.
Table of Contents
Introduction
Functions in SQL Server are the essential building blocks of executing reusable logic and computations inside a database. They enable developers to encapsulate code, facilitating code management, maintenance, and reuse across different application components. By utilizing functions, you may enforce consistency, expedite complex searches, and enhance the overall efficiency of your database operations.
A Glimpse into the History
Over time, SQL Server’s notion of functions has changed significantly. Functions first included in the early editions of SQL Server have become more sophisticated and valuable due to the growing demands of contemporary data processing. They started as straightforward procedures for encapsulating logic but have developed into solid instruments capable of managing anything from straightforward computations to intricate data transformations.
What Do SQL Server Functions Mean?
Database objects that take arguments carry out an operation (such as a computation or data transformation), and then return a result are called functions in SQL Server. Although they are a stored procedure, their purpose is to provide a table or value in return. Queries include the ability to call functions, which lets you directly apply logic to data sets.
Advantages of Functions in SQL Server
A few advantages of using functions & different types of functions in SQL Server are given below for more clarity & better understanding:
Reusability of Code
Description: Functions are a useful tool for encapsulating logic that can be applied to various queries and stored processes.
Benefit: Reducing code duplication improves the maintainability and ease of updating your SQL scripts.
Adjustability
Description: Functions allow developers to develop SQL modularly, breaking down large tasks into smaller, more manageable chunks.
Benefit: This makes the codebase more organized and easier to understand, especially in complex systems.
Consistency
Description: By centralizing logic in functions, you ensure that the same logic is implemented uniformly across the program.
Benefit: Because the logic only needs to be changed once, there is a lower chance of mistakes and inconsistencies.
Simplification of Complex Queries
Description: Functions help simplify complex SQL queries by dividing intricate tasks into smaller, more manageable chunks.
Benefit: This makes the SQL code easier to maintain and read, facilitating optimization and troubleshooting.
Optimizing Performance
Description: By eliminating pointless calculations or actions that would otherwise be repeated across several queries, well-designed functions can enhance query performance.
Benefit: Quicker query execution times and more economical resource use may result from this.
Testing and debugging made it easy
Description: Functions, particularly scalar and table-valued functions, can be verified independently of the primary application logic.
Benefit: This allows for more detailed testing and finding problems with individual logic blocks more quickly.
Disadvantages of Functions in SQL Server
A few disadvantages of using functions & different types of functions in SQL Server are given below for more clarity & better understanding:
Possible Overhead in Performance
Description: Significant performance loss can result from the overuse of functions, particularly those that are sophisticated or called within huge datasets.
Benefit: Because functions are frequently run row by row, they might cause query execution to lag, especially when scalar functions are employed in SELECT statements.
Restricted Error Management
Description: SQL Server functions’ error-handling capabilities are somewhat restricted compared to stored procedures.
Benefit: This restriction may make handling exceptions and unforeseen circumstances more challenging, weakening the code’s resilience.
Limitations on Adverse Reactions
Description: Operations like INSERT, UPDATE, and DELETE that change the state of the database cannot be carried out by functions.
Benefit: This constraint makes functions less flexible, especially when data manipulation is required.
Analyzing Complexity
Description: Debugging functions can be difficult, particularly if they contain a lot of complicated logic or are heavily integrated into other queries.
Benefit: Tracking and fixing problems may be challenging because SQL Server functions lack direct debugging capabilities.
Handling Dependencies
Description: Functions, especially those used repeatedly, can lead to dependencies in your SQL codebase.
Benefit: Modifications to a function may have broad implications, necessitating thorough testing to guarantee that all dependant code operates as intended or perhaps introducing flaws.
Problems with Concurrency
Description: If a function is not carefully developed, it may generate concurrency concerns, especially if it involves sophisticated calculations or data retrieval.
Benefit: In a multi-user environment, poorly optimized routines may result in locks or blocking, which can cause performance bottlenecks.
Types of Functions in SQL Server
Functions & different types of functions in SQL Server are grouped according to the kind of output they yield and how they are used. An outline of the primary SQL Server functions & different types of functions are provided below:
Functions of Scalars
A single value of a particular data type, such as an integer, string, or date, is returned by scalar functions.
Use Case: Frequently employed in SQL queries for computations, data type conversions, and string manipulation.
Example of types of functions:
CREATE FUNCTION dbo.CalculateProductOfNumber (@Num INT)
RETURNS INT
AS
BEGIN
RETURN Num * Num;
END;
Table-Valued Functions (TVFs)
Table-valued functions yield a table data structure that may be queried like a conventional table. When you need to return rows that may be filtered, combined, or aggregated, they come in handy.
Categories:
Linear Functions Valued by Tables
Like a view, but with the ability to accept parameters, all these functions do is return a table with a single SELECT command.
Example of types of functions:
CREATE FUNCTION dbo.FetchStudentByClassID (@ClassID INT)
RETURNS TABLE
AS
RETURN
(
SELECT RollNo, StudentName, ClassID
FROM Students
WHERE ClassID = @ClassID
);
Table-Valued Multi-Statement Functions:
It is more intricate than inline TVFs, enabling the creation of the final table using several SQL statements. These functions feature a BEGIN…END block that allows for executing different operations.
Example of types of functions:
CREATE FUNCTION dbo.GetOrders (@From DATE, @To DATE)
RETURNS @OrderSummary TABLE
(
OrderID INT,
TotalOrderAmount DECIMAL(15, 2)
)
AS
BEGIN
INSERT INTO @OrderSummary
SELECT OrderID, SUM(TotalOrderAmount)
FROM Orders
WHERE OrderDate BETWEEN @From AND @To
GROUP BY OrderID;
RETURN;
END;
Aggregate Functions
Aggregate functions calculate a single value from a set of values. They are pre-built routines that calculate statistics across a range of rows.
Typical Aggregate Tasks:
MAX(): Determines a set’s maximum value.
MIN(): Determines a set’s minimum value.
SUM(): Determines a numeric column’s total sum.
AVG(): Determines a numeric column’s average value.
COUNT(): Gives back the number of rows in a set.
Example of types of functions:
SELECT COUNT(*) AS [NoOfStudents] FROM Students;
SELECT AVG(Salary) AS [AverageSalary] FROM Employees;
SELECT MAX(Salary) AS [HighestSalary] FROM Employees;
SELECT MIN(Salary) AS [LowestSalary] FROM Employees;
SELECT SUM(OderAmount) AS [TotalOrderValue] FROM Orders;

System Functions
Description: SQL Server pre-defined functions that carry out a range of system-related operations. These functions cover Numerous tasks, such as retrieving metadata, manipulating dates and times, and string processing.
Typical System Features:
GETDATE(): Provides the time and date as of right now.
LEN(): Gives back a string’s length.
ISNULL(): This function substitutes a given replacement value for NULL.
Use CAST and CONVERT functions to convert data between different types.
Example of types of functions:
SELECT GETDATE() AS [CurrentDate&Time];
SELECT LEN('Microsoft SQL Server is a Relational Database Management System & is used by many organizations in the world.') AS [LengthOfLine];
SELECT CAST('7253' AS varchar) AS int
SELECT CONVERT (VARCHAR, '7253')
Functions of Windows
Description: Window functions compute values for the current row across a range of table rows. In contrast to aggregate functions, window functions do not combine the result set into a single output row.
Typical Window Features:
ROW_NUMBER(): Gives each row in a result set’s partition a distinct sequential integer.
RANK(): Gives a rank to every row in a partition, leaving spaces in the ranking for ties.
SELECT
ItemID,
OrderAmount,
RANK() OVER (ORDER BY OrderAmount DESC) AS OrderAmountRank
FROM Orders;
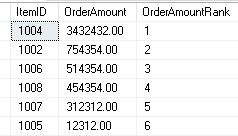
Functions of System Statistics
These routines offer details regarding the data distribution statistics in tables or indexes.
Typical Statistical Functions of the System:
STATS_DATE() returns the most recent statistics update date for the given index or statistics object.
Example of types of functions:
SELECT STATS_DATE(object_id('Orders'), 1) AS StatsDate;

These kinds of SQL Server functions provide a wide range of capabilities, enabling you to carry out simple math operations and intricate data transformations and manipulations, thus improving the functionality and efficiency of your SQL queries.
Performance Advice for SQL Server Functions
Optimizing SQL Server functions is essential to sustaining effective database performance. The following are some general performance pointers when using SQL Server functions:
Refrain from Using Scalar Functions in Select Clauses
Why: Scalar functions are frequently performed for each row the query returns, resulting in row-by-row processing, which can significantly reduce performance when used in SELECT clauses.
Advice: To reduce performance overhead, consider replacing scalar functions with table-valued or inline logic.
Make use of iTVFs or inline table-valued functions
Why: Because ETFs integrate the function’s logic into the query execution, they enable SQL Server to optimize the query plan and frequently produce better results than scalar functions or multi-statement TVFs.
Advice: Use iTVFs instead of other function types, mainly when querying massive datasets.
Maintain Operations Lightweight and Uncomplicated
Why: Complex functions can result in longer execution times and inefficient query plans.
Advice: Reduce the number of needless calculations and operations by streamlining the logic inside functions. If necessary, divide difficult jobs into smaller, more doable ones.
Reduce Internal Data Access for Functions
Why: Querying big tables or doing joins inside functions are examples of excessive data access impeding performance.
Restrict the quantity of data that the function can access. Stored procedures can be a better option if significant data processing is required.
Employ Relevant Data Types
Why: Using the appropriate data types can avoid needless data conversions, which can hurt speed.
Advice: Use the most minor and proper data types for function parameters and return values.
Steer clear of recursive functions.
Why: Recursive functions can use a lot of CPU and memory, especially when the recursion depth is vast or unbounded.
Advice: To lower the chance of performance problems, rebuild recursive procedures whenever possible using iterative techniques.
Indexes of Leverage
Why: Well-executed indexing can significantly boost the efficiency of data retrieval operations.
Avoid utilizing functions on columns that are part of a WHERE clause or join condition, as this can prevent SQL Server from efficiently employing indexes. Instead, make sure that tables accessible within functions are adequately indexed.
Keep an eye on function performance.
Why: Frequent monitoring aids in locating function-related performance bottlenecks.
Advice: To track function performance and make necessary optimizations, use SQL Server Profiler, Extended Events, or Dynamic Management Views (DMVs).
Keep Functions Out of WHERE Clauses
Why: Because functions in WHERE clauses may cause SQL Server to utilize indexes less effectively, they may result in full table scans.
Advice: If feasible, compute the function value before the WHERE clause or employ different data filtering methods.
Consistently Test Function Performance
Why: Over time, variations in data volume or usage habits may affect the effectiveness of some operations.
Advice: Test functions regularly to guarantee they continue operating at their best. It is essential after major database updates or modifications.
Examine Other Options for Functions
Why: Functions may not always be the most excellent tool for the job, particularly when handling transactions or requiring extensive data processing.
A stored procedure, view, or other SQL construct may be more effective for your use case. Consider this while making your decision.
Turn on SET NOCOUNT
Why: By stopping DONE_IN_PROC messages from being sent to the client after each statement, the SET NOCOUNT ON statement can enhance performance.
Including SET NOCOUNT ON at the start of your function can reduce unnecessary network traffic and enhance efficiency.
Examples of functions in SQL server
SQL Server provides a multitude of features, each with a distinct purpose. Here are some instances of various SQL Server function types:
SQL Server’s Scalar Functions
You can use scalar functions anywhere an expression is legal and return a single value, such as a number, text, or date.
Example: Scalar Function to find student-wise age
CREATE FUNCTION dbo.CheckStudentAge (@StudentName Varchar(100),@BirthDate DATE)
RETURNS varchar(150)
AS
BEGIN
RETURN 'The '+@StudentName+' is now '+CONVERT(VARCHAR, (DATEDIFF(YEAR, @BirthDate, GETDATE()) -
CASE
WHEN MONTH(@BirthDate) > MONTH(GETDATE()) OR
(MONTH(@BirthDate) = MONTH(GETDATE()) AND DAY(@BirthDate) > DAY(GETDATE()))
THEN 1
ELSE 0
END))+' years old.'
END
go
Usage:
SELECT dbo.CheckStudentAge('1985-08-15') AS [Student Wise Age];
SQL Server’s Inline Table-Valued Functions (iTVFs)
When a query returns several rows and columns, inline table-valued functions are helpful because they return a table data type.
Example: Inline Table-Valued Function to Get Order by ItemID
CREATE FUNCTION dbo.GetOrderDetailsByItemID (@ItemID INT)
RETURNS TABLE
AS
RETURN
(
SELECT [OrderID]
,[ClientID]
,[OrderDate]
,[OrderAmount]
,[ItemID]
FROM [mOrders].[dbo].[Orders]
WHERE [ItemID] = @ItemID
)
Usage:
SELECT * FROM dbo.GetOrderDetailsByItemID(1004);
SQL Server’s Multi-Statement Table-Valued Functions (mTVFs)
Table-valued functions with multiple statements enable filling a table variable with numerous statements before its return.
Example: Multi-Statement Table-Valued Function to Get Sales Summary
CREATE FUNCTION dbo.GetOrderSummary (@OrderStartDate DATE, @OrderEndDate DATE)
RETURNS @OrderSummary TABLE
(
ClintID INT, ItemID int,
OrderAmount DECIMAL(18, 2)
)
AS
BEGIN
INSERT INTO @OrderSummary
SELECT ClientID, ItemID, SUM(OrderAmount) AS [Order Amount]
FROM Orders
WHERE OrderDate BETWEEN @OrderStartDate AND @OrderEndDate
GROUP BY ClientID, ItemID
Return;
END
Usage:
SELECT * FROM dbo.GetOrderSummary('2023-01-01', '2024-03-31');
SQL Server Aggregate Functions
Aggregate functions compute a single value after applying a set of values.
Example: Using Built-in Aggregate Function SUM
SELECT ClientID, ItemID, SUM(OrderAmount) AS [Order Amount]
FROM Orders
GROUP BY ClientID, ItemID
SQL Server System Functions
SQL Server has built-in system functions that allow you to work with data and information.
Example: Using System Function GETDATE to Get the Current Date and Time
SELECT GETDATE() AS [Current Date Time];
Example: Using System Function NEWID to Generate a Unique Identifier
SELECT NEWID() AS [New Unique ID];
SQL Server’s Built-in String Functions
These scalar functions are made to work with string data.
Example: Using UPPER and LEN Functions
SELECT UPPER(StudentName) AS [Student Name In Caps Letter],
LEN(StudentName) AS [Length Of Student Name]
FROM Employees;
SQL Server’s Built-In Date Functions
Functions for working with date and time data are available in SQL Server.
Example: Using DATEADD and DATEDIFF
SELECT DATEADD(DAY, 7, GETDATE()) AS [Next Week Date],
DATEDIFF(DAY, '2024-01-01', GETDATE()) AS [No. Of Days Since Current Year];
Conclusion
Your database applications’ performance, reusability, and flexibility can all be improved with the help of SQL Server functions. SQL code can be written more effectively and maintainable if you know the many kinds of functions, their benefits and drawbacks, and how to use them. SQL Server functions are an indispensable component of your toolkit, regardless of the complexity of the data transformations you create or the simple calculations you do.
FAQs
Q: What is the primary function of a SQL Server function?
Ans: Functions simplify complicated tasks by encapsulating logic that may be reused across several queries.
Q: Can SQL Server operations change data?
Ans: No, SQL Server functions cannot change the state of the database.
Q: What sets table-valued functions apart from scalar functions?
Ans: Table-valued functions return a data table, whereas scalar functions return a single result.
Q: What’s a Table-Valued Inline Function?
Ans: It’s a function that takes parameters and returns a table, much like a view.
Q: Do functions perform better than processes that are stored?
Ans: Efficiency is use case-specific. Functions can improve performance in some cases; nevertheless, if they are abused, they can result in overhead.
Q: Is it possible to employ functions inside a select statement?
Ans: Yes, functions frequently apply logic to data in SELECT statements.
Q: What do SQL Server aggregate functions do?
Ans: Aggregate functions compute a single result, like SUM or AVG, based on a set of variables.
Q: How do user-defined functions and system functions differ from one another?
Ans: While users create user-defined functions to address particular needs, SQL Server already includes system functions.
Q: In a function, what does the keyword RETURNS mean?
Ans: The function’s data type or table structure that will use the RETURNS keyword specifies the output.
Q: What is the maximum number of parameters a function can take?
Ans: Complex operations are made possible by functions’ ability to take numerous parameters.
Review the below articles also
SQL Managed Instance: Faster & Improved
TSQL Database Backup: Top 7 Usage
Decommission A Server: In 6 Easy Steps
Discover Recovery Model in SQL Server